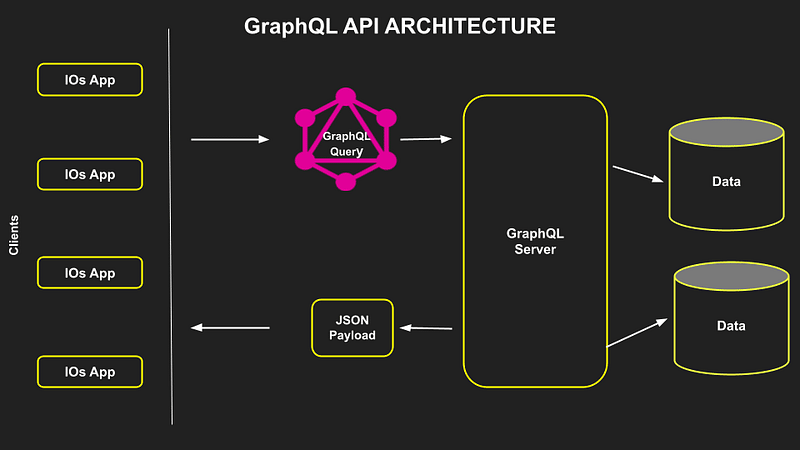
1. What is GraphQL and why do we need it?
GraphQL, developed by Facebook, is a revolutionary query language for APIs. Unlike traditional REST APIs, GraphQL allows clients to request precisely the data they need, minimizing over-fetching and under-fetching(reducing unnecessary data retrieval and ensuring all needed data is fetched efficiently.). The need for GraphQL arises from the shortcomings of REST in providing flexibility and efficiency in data retrieval.
2. What issues do we face in REST API in terms of response?
REST APIs often suffer from issues like over-fetching, where clients receive more data than necessary, leading to bandwidth waste, and under-fetching, requiring additional requests for complete data retrieval. GraphQL addresses these problems by enabling clients to specify their data requirements in a single request.
In a REST API, when a client requests information about a specific blog post, it might receive more details than needed. For instance, if the response includes the entire user profile for the author of the post, but the client only requires the author’s name, this would be an example of over-fetching.
// Over-fetching in REST response
{
"postId": 123,
"title": "Exploring REST Over-fetching",
"content": "Lorem ipsum...",
"author": {
"id": 456,
"username": "john_doe",
"email": "[email protected]",
"avatar": "url_to_avatar"
// ... other unnecessary details
}
}
Additionally, if the client needs information about the post’s comments, it may have to make a separate request to another endpoint, leading to under-fetching.
In contrast, with GraphQL, the client can specify its exact data requirements in a single query. If the client only needs the post title and the author’s username, it can request just that, eliminating over-fetching.
// GraphQL query to eliminate over-fetching
query {
post(id: 123) {
title
author {
username
}
}
}
Furthermore, if the client wants information about the post’s comments, it can include that in the same query, preventing the need for additional requests and addressing the issue of under-fetching.
// GraphQL query to include comments
query {
post(id: 123) {
title
author {
username
}
comments {
id
text
}
}
}
This demonstrates how GraphQL enables clients to precisely define their data needs, avoiding unnecessary data transfer and additional requests, thereby optimizing the efficiency of data retrieval.
3. Comparison between REST and GraphQL
- Flexibility: GraphQL offers fine-grained control over data retrieval, preventing over-fetching and under-fetching.
- Single Request: GraphQL allows clients to fetch all required data in a single request, reducing round trips compared to REST.
- Versioning: GraphQL supports backward-compatible changes without versioning, unlike REST, which may require version updates.
- Discoverability: GraphQL’s self-documenting schema simplifies API exploration and eliminates the need for separate documentation.
4. Explaining Basic Terms of GraphQL
- Type: In GraphQL, a type represents a structured set of data. For example, a “Task” type could have fields like “id” and “title.”
- Query: A query is a request for specific data. Clients use queries to specify the exact information they need.
- Mutation: Mutations are operations that modify data. In a TODO app, a mutation might be used to add or remove a task.
- Subscription: Subscriptions enable real-time updates. Clients can subscribe to changes and receive notifications when data changes on the server.
- Input: Input types are used as arguments for mutations. They define a set of parameters a client can pass to modify data.
- Data Types: GraphQL supports various data types, including scalar types (int, float, string, boolean) and complex types (objects, enums, interfaces).
5. GraphQL installation with Apollo Server in Node.js
To harness the power of GraphQL with Node.js, we turn to Apollo Server. Begin by installing the necessary packages:
npm install apollo-server graphql
6. Create a Simple TODO app with GraphQL Schema and Resolvers
6.1 Define the GraphQL Schema:
type Task {
id: ID!
title: String!
}
type Query {
tasks: [Task!]!
}
type Mutation {
addTask(input: TaskInput!): Task!
removeTask(id: ID!): Task!
}
input TaskInput {
title: String!
}
6.2 Implement Resolvers:
const tasks = [];
let taskId = 1;
const resolvers = {
Query: {
tasks: () => tasks,
},
Mutation: {
addTask: (_, { input }) => {
const newTask = { id: taskId++, title: input.title };
tasks.push(newTask);
return newTask;
},
removeTask: (_, { id }) => {
const removedTask = tasks.find(task => task.id === id);
tasks = tasks.filter(task => task.id !== id);
return removedTask;
},
},
};
module.exports = resolvers;
Here is the final code of index.js, you can put the GraphQL typedefs and resolvers on appropriate position.
const { ApolloServer, gql } = require("apollo-server");
const typeDefs = gql`
# Put GraphQL Schema Here
type Query {
count: Int!
}
`
const resolvers = {
// put resolvers here
Query: {
count: () => 0
}
}
const server = new ApolloServer({
typeDefs,
resolvers
})
server.listen(4000)
.then(({ url }) =>
console.log(`Server started at: ${url}`)
)
Once you complete the above steps then run node index.js and then open localhost:4000, you will see the following window, you can click on Query Your Server to start working on the GraphQL dashboard
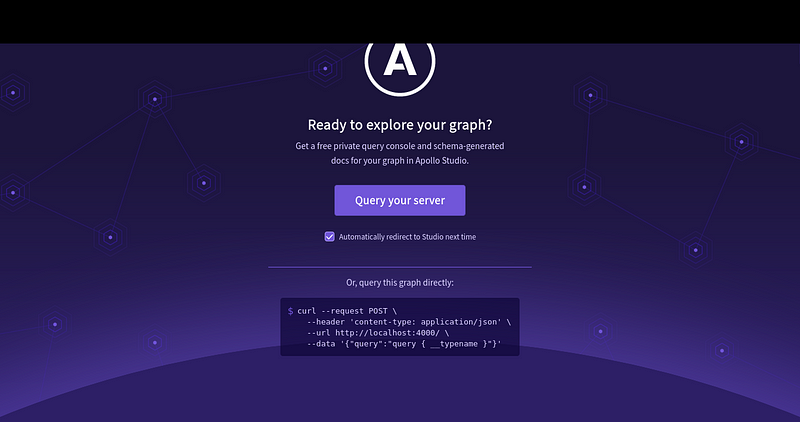
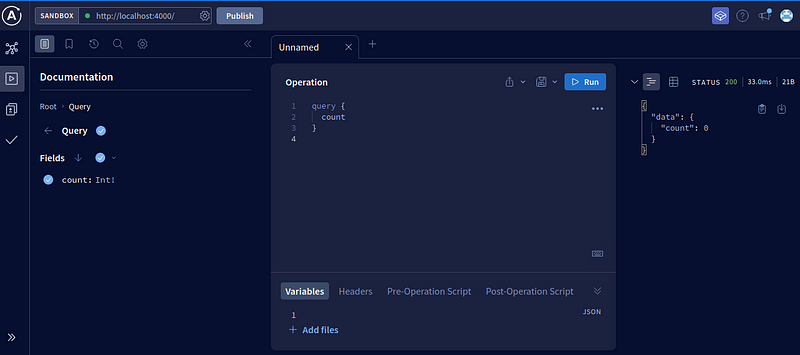
7. Test the TODO App on GraphQL Page
Open your GraphQL playground (usually at http://localhost:4000
) and try the following queries:
query {
tasks {
id
title
}
}
mutation {
addTask(input: { title: "Complete Blog" }) {
id
title
}
}
mutation {
removeTask(id: 1) {
id
title
}
}
8. Conclusion and Scope of GraphQL
In conclusion, GraphQL empowers developers by providing a flexible and efficient means of building APIs. Its ability to address the limitations of REST, coupled with real-time capabilities through subscriptions, positions GraphQL as a key player in the future of web development. As the tech landscape evolves, the scope of GraphQL is likely to expand, making it an essential tool for developers looking to build scalable and dynamic applications. Embrace GraphQL and unlock a new era of API development!