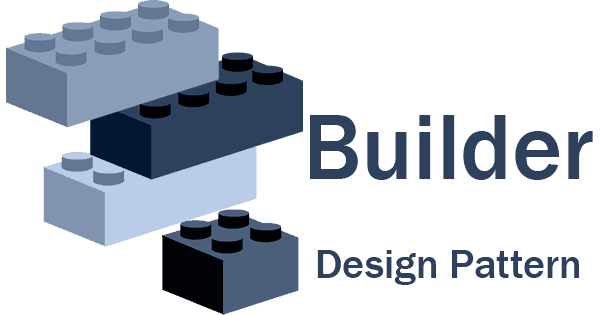
What is builder design pattern?
The Builder design pattern is a creational design pattern that provides a way to construct complex objects step by step. It separates the construction of an object from its representation, allowing the same construction process to create different representations. The pattern is useful when you want to create an object with many optional parameters or configurations.
Why do we need builder design pattern?
The Builder design pattern is useful in several scenarios:
- Creation of complex objects: If an object requires a large number of parameters or configurations to be set during its creation, using a constructor with numerous parameters can become unwieldy and error-prone. The Builder pattern allows you to construct the object step by step, providing a clean and readable way to specify the required parameters and optional configurations.
- Object configuration variations: When you need to create multiple variations of an object with different configurations, using the Builder pattern allows you to reuse the construction process while altering the parameters and configurations. This reduces code duplication and provides a consistent and predictable way to create different object instances.
- Encapsulation of construction logic: The Builder pattern separates the construction logic from the actual object, encapsulating it within the builder class. This separation helps to improve code readability, maintainability, and reusability. It allows you to modify or extend the construction process without affecting the client code that uses the builder.
- Fluent interface and method chaining: The Builder pattern often employs a fluent interface, where the builder methods return the builder instance itself. This enables method chaining, allowing you to chain multiple method calls in a concise and readable manner. It makes the code more expressive and facilitates the step-by-step construction process.
- Creation of immutable objects: Builders can be used to create immutable objects, where the object’s state cannot be modified after its creation. By setting the object’s properties during the construction process, the builder can ensure that the resulting object is immutable and thread-safe. Overall, the Builder pattern provides a flexible and scalable way to construct complex objects while improving code readability, maintainability, and reusability. It is particularly useful when dealing with objects with multiple parameters or configurations and when creating different variations of objects
Here’s an example of how the Builder pattern can be implemented in Python:
class Pizza:
def __init__(self):
self.crust = None
self.sauce = None
self.toppings = []
def __str__(self):
return f"Crust: {self.crust}, Sauce: {self.sauce}, Toppings: {', '.join(self.toppings)}"
class PizzaBuilder:
def __init__(self):
self.pizza = Pizza()
def set_crust(self, crust):
self.pizza.crust = crust
return self
def set_sauce(self, sauce):
self.pizza.sauce = sauce
return self
def add_topping(self, topping):
self.pizza.toppings.append(topping)
return self
def build(self):
return self.pizza
# Usage
builder = PizzaBuilder()
pizza = builder.set_crust("thin").set_sauce("tomato").add_topping("cheese").add_topping("pepperoni").build()
print(pizza)
In the example above, we have a Pizza class that represents a pizza object. The PizzaBuilder class is responsible for constructing the pizza step by step. It has methods like set_crust, set_sauce, and add_topping to set the desired properties of the pizza. Each of these methods returns the builder instance itself, allowing method chaining.
Finally, the build method is called to retrieve the constructed pizza object. This method returns the fully constructed Pizza object. By using the Builder pattern, we can create a pizza with different configurations by specifying the desired crust, sauce, and toppings. The pattern provides a clear and flexible way to construct complex objects while keeping the construction process separate from the object itself.
In conclusion, the Builder design pattern is a creational pattern that separates the construction of complex objects from their representation. It provides a step-by-step approach to create objects with multiple parameters or configurations, allowing for flexibility and reusability.
The Builder pattern is beneficial in scenarios where objects require a large number of parameters, different configurations, or variations. By encapsulating the construction logic within the builder class, it improves code readability, maintainability, and allows for easy modification or extension of the construction process.
The pattern’s fluent interface and method chaining enable a concise and expressive way to specify the object’s properties. It also facilitates the creation of immutable objects, ensuring thread-safety and preventing modification of the object’s state after construction.
Overall, the Builder pattern offers a structured and organized approach to construct complex objects, providing a clear separation between construction and representation, and making the code more maintainable and flexible.
Thanks for reading this blog hope you have liked it. If yes then please clap and follow me for more blogs like this.
Did you enjoy this article? If so, get more similar content by subscribing to Decoded, our YouTube channel!. Thanks for reading. Happy coding.
The same blog will also be available on https://ajaykrp.me/