
What is TypeScript?
As you know, JavaScript is not a strongly typed language. So TypeScript used to add static type on top of JavaScript. TypeScript is a syntactic superset of JavaScript which adds static typing.
This basically means that TypeScript adds syntax on top of JavaScript, allowing developers to add types.
Why to use TypeScript?
JavaScript is a loosely typed language. It can be difficult to understand what types of data are being passed around in JavaScript.
In JavaScript, function parameters and variables don’t have any information. So developers need to look at documentation, or guess based on the implementation.
TypeScript allows specifying the types of data being passed around within the code, and has the ability to report errors when the types don’t match.
TypeScript Project Setup
First initialize a NodeJS project with the following command
npm init -y
Now install typescript as dev dependency
npm install typescript --save-dev
You can verify whether typescript is installed or not by typing following command. By running following command you will get typescript version installed in your system.
npx tsc --version
Now you have to initiate typescript project by typing following command
npx tsc --init
Above command will generate tsconfig.json
file which will specifies the root files and the compiler options required to compile the project. You can learn more about this file here.
Install Required Npm Dependencies
Since we are going to use express to develop rest API so let’s install express first.
npm install --save express
npm install --save-dev @types/express
Here i have installed express for typescript as well which is required to install to use in the typescript application. If you don’t install @types/express then you will get package not found error while importing express in the application.
Now install ts-node-dev
which is used to watch changes in the application and restart automatically whenever we make any change. This works same as nodemon
. You can learn more about this package here.
After all above installation your project.json
will look something like this
{
"name": "tsc_tuts",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "ts-node-dev --respawn --transpile-only app.ts"
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"ts-node-dev": "^2.0.0",
"typescript": "^4.9.3"
},
"dependencies": {
"@types/express": "^4.17.14",
"express": "^4.18.2"
}
}
Please note the start command here i have added command suggested by in the node-dev
documentation. You can refer it i have mentioned link above.
Update app.js
file with the following code
import express from 'express';
const app = express();
const PORT = 3000;
app.get("/", (req, res) => {
res.send({message: "Hello World!"});
});
app.listen(PORT, () => {
console.log(`Server is listening to port: ${PORT}`);
})
Here our application is running on the 3000 port. Try running project with npm start
command. Now you can open http://localhost:3000/ . You will get following output

Build one simple task API with MVCS architecture
Now create three folders controllers
, routes
and services
for the Api. Now your folder structure will look something like this.
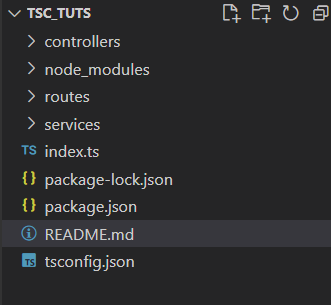
Now add task.route.ts
in the routes
folder.
// task.route.ts
import express from "express";
import { TaskController } from "../controllers";
const router = express.Router();
router.route('/task').post(TaskController.createTask);
export default router;
If you can notice i have imported task controller from the controllers folder. So we have to create controller as well. So now let’s create task.controller.ts
and index.ts
// task.controller.ts
import { Express, Request, Response, NextFunction } from "express"
import { TaskService } from "../services";
const createTask = async (req: Request, res: Response, next: NextFunction) => {
const task = TaskService.createTask();
res.json({ status: 'success', task });
};
export default { createTask };
Here in the async function as normal we have passed three parameters but one thing to notice here. I have added type of that parameters. For req
i have added type Request, for res
added type Response and for next
function added type NextFunction. And this is the main use case of typescript to define type of variables or objects.
In typescript types are defined in two ways
- using
type
// type declaration
type Person = {
name: string,
age: number
}
// example 1
const person1: Person = {name: 'ajay', age: 25};
// example 2
const person2: Person = {name: 'ajay', age: "25"}; // will show following error
// Error Type 'string' is not assignable to type 'number'.ts(2322)
2. Using interface
interface Person {
name: string,
age: number
}
// example 1
const person1: Person = {name: 'ajay', age: 25};
// example 2
const person2: Person = {name: 'ajay', age: "25"}; // will show following error
// Error Type 'string' is not assignable to type 'number'.ts(2322)
So here we have seen how to assign type to object. The same way you can assign type to variables as well. But one thing you have to remember you can not assign type which is created using interface, you can only assign type which is created using type keyword. This is the one of difference in type and interface.
Now lets create index.ts
for controller to access it directly from the controllers
folder
// index.ts
export {default as TaskController} from './task.controller';
If you notices in the task.controller.ts file we are using TaskService. So now lets create task.service.ts
and index.ts
// task.service.ts
const createTask = () => {
// write your logic here. You can write all DB related operations here and any third party connections also
return { name: 'New Task' };
}
export default { createTask };
// index.ts
export {default as TaskService} from './task.service';
Now add task
route to app.ts
and prepend /api/v1
to the api which we have created. So now our updated app.ts
file will look something like this.
import express from 'express';
// added this line
import taskRoute from "./routes/task.route";
const app = express();
const PORT = 3000;
app.get("/", (req, res) => {
res.send({ message: "Hello World!" });
});
// added this line
app.use("/api/v1", taskRoute);
app.listen(PORT, () => {
console.log(`Server is listening to port: ${PORT}`);
})
Now you can run project using npm start
command. To test API is working or not you can use postman or curl. I am adding curl command here
curl -XPOST http://localhost:3000/api/v1/task
you will get following output
{
"status": "success",
"task": {
"name": "New Task"
}
}
Conclusion
In this blog we have seen how we can integrate typescript to NodeJS application. Here i have build simple task API using MVCS architecture.
Thanks for reading this blog hope you have liked it. If yes then please clap and follow me for more blogs like this. I have my own YouTube channel where i create NodeJS based projects, you can check it out here https://shorturl.at/LMU48. The same blog will also be available on https://ajaykrp.me/