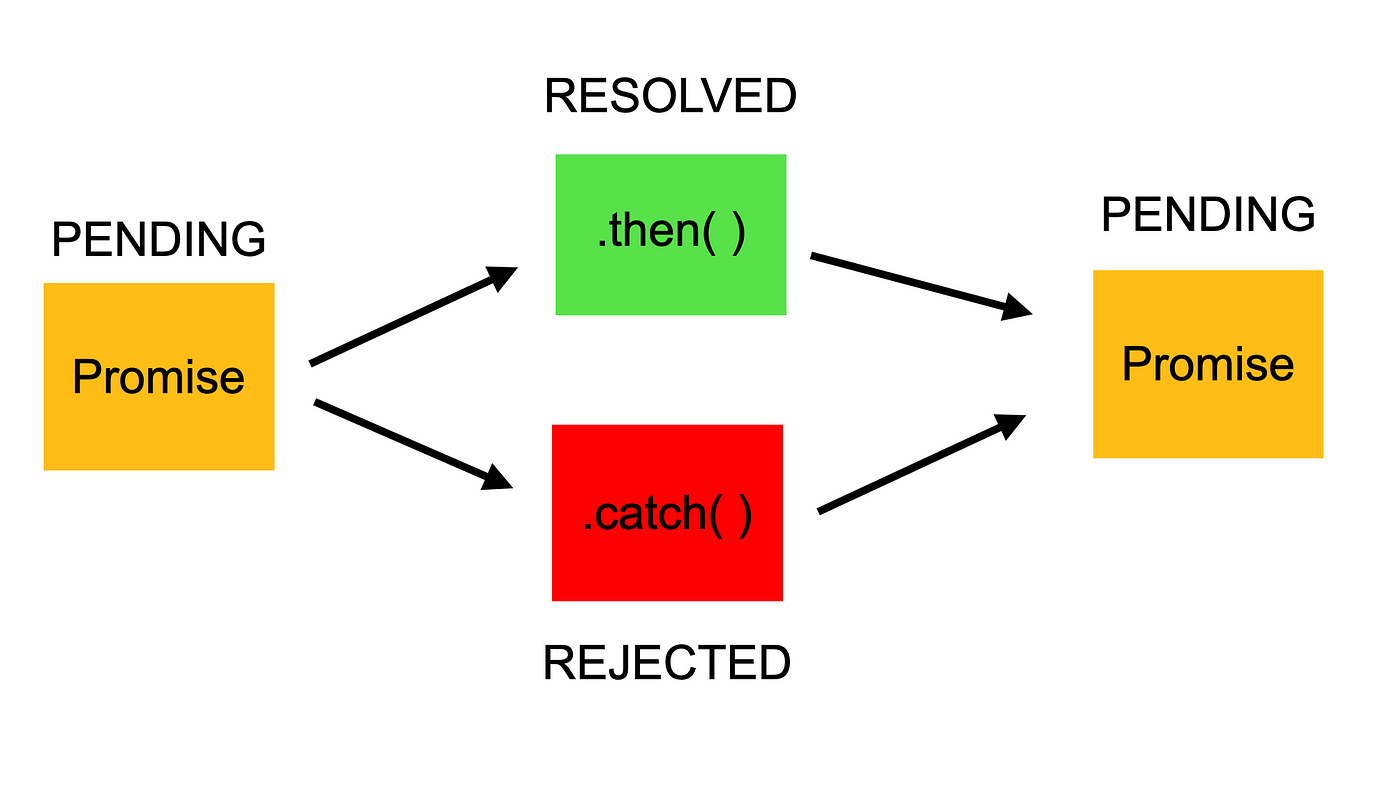
Promise allow you to deal with eventual value or errors or in other words it allows you to deal with the future(asynchronous) value(IO blocking tasks value). For example network calls, reading files, etc. This lets asynchronous methods return values like synchronous methods: instead of immediately returning the final value, the asynchronous method returns a promise to supply the value at some point in the future.
Promise has following set of states
- pending — Initial state, neither fulfilled nor rejected.
2. fulfilled: meaning that the operation was completed successfully.
3. rejected: meaning that the operation failed.
Syntax of Promise
const promise = new Promise((resolve, reject) => {}).then(out => {}).catch(err => {})
Promise takes two parameter as arguments, one is resolve and another one is reject. resolve is called when the future(IO blocking tasks) are done without any error then you can call resolve with the output of the IO tasks values.
While reject is called when you get some error while performing some blocking tasks.
Chained Promise & reject bypass
We can create chain of promise with .then function. In the then function we can pass values to the next then function. we can also throw errors in the then function which will bypass all then next to current then function and catch error in cache function.
const promise = new Promise((resolve, reject) => {
// Both asynchronous or synchronous code can be present here
resolve('good')
//reject('bad') // this will directly call catch method
})
.then(data => {
console.log(data);
return 1;
})
.then(data => {
throw 'really bad'; // this exception will get caught in the catch method and next then function will get bypass
console.log(data);
return 2;
})
.then(data => {
console.log(data);
return 3;
})
.then(data => {
console.log(data);
return 4;
})
.catch(err => {
console.log(err);
})
Advantages of Promise
- To overcome callback hell we can use Promise
To overcome callback hell we can use promise .then -> .then -> .then as we require methods to make it behave like synchronous code
const fs = require('fs');new Promise((resolve, reject) => {
fs.readFile('../dir/file1.txt', (err, data) => {
if (err) {
reject(err);
} else{
resolve(data.toString());
}
})
}).then(data => {
console.log(data);
}).catch(err => {
console.log(err);
})
2. Call multiple synchronous methods at once
We can call multiple synchronous functions at once using promise.all function. Here i am taking example of reading files from disk-
To overcome reading files in callback hell manner we can use promise.all method and output of all files will be available in the array format in nearest .then method.
Promise.all method takes a argument as a array of promises which we have extracted from the util.promisify method
const fs = require('fs');
const utils = require('util');const read = utils.promisify(fs.readFile);
Promise.all([
read('../dir/file1.txt'),
read('../dir/file2.txt'),
read('../dir/file3.txt')
]).then(
data => {
console.log(data[0].toString());
console.log(data[1].toString());
console.log(data[2].toString());
})
That’s all i have to share on promise. If you have more points to share please comment down below. It will help me and others to gain more knowledge on promise. Thanks for reading. Please follow to support and more contents like this.