
Data types are divided into two groups in JavaScript
- Primitive data types — It is a data that is not an object and has no methods or properties.
All primitives are immutable; that is, they cannot be altered. The variable may be reassigned to a new value, but the existing value can not be changed.
2. Non-primitive/Reference Data type — are Objects and Arrays. Reference types in JavaScript are mutable, meaning that the state and fields of mutable types can be changed. No new instance is created as a result.
Object mutation in Javascript
Few days back i was trying to copy one object and modify the copied Object. What i saw was, the original object was also getting updated when i was trying to update copied object. And this is called object mutation. Let me tell you how mutation works. When you copy any object it take reference of original object which means copied object is pointing to the original object and that’s the reason it was updating the original object. You can understand it with the following diagram

Now lets understand this with the example
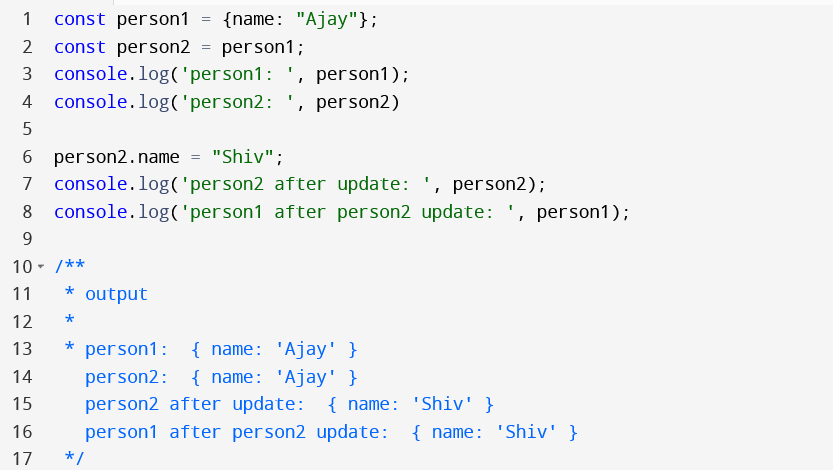
Lets understand code line by line
Line1: here we have declared one object person1 and assigned one object with name as key.
Line2: here we have declared second object person2 and assigned person1 object to it.
Line3 & line4 we checked object by console log and got expected value.
Line6: Here we are trying to update person2 object name with value “Shiv”.
line7: Here we again console log person2 and got person2 value as expected.
line8: Here real magic happened. The expected output as we were thinking it should be {name: “Ajay”} but we got {name: “Shiv”}.
Why this happened? When we updated person2, person1 also got changed and this is happening because of memory reference(person2 updated the original reference so all object which is pointing to this reference will get updated) or you can say object mutation which i have explained with diagram.
How to prevent Object mutation?
There are many third party libraries available which we can use to prevent object mutation. But here i am going to explain 2 JavaScript technique
1. Object.assign()
This method tried to copy all parameters from source object to destination object. This method can give mutable object as output if not used properly. It’s easy to avoid mutating objects by providing an empty object in the target parameter.
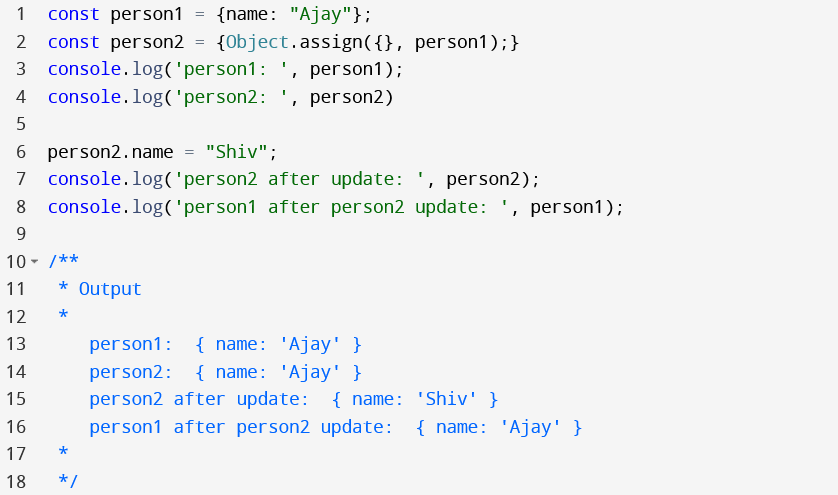
Here on line2 we have used Object.assign method and this method gives us output as expected.
2. Spread Syntax
JavaScript spread syntax can be used to prevent object mutation. You can lean more about spread syntax from here.
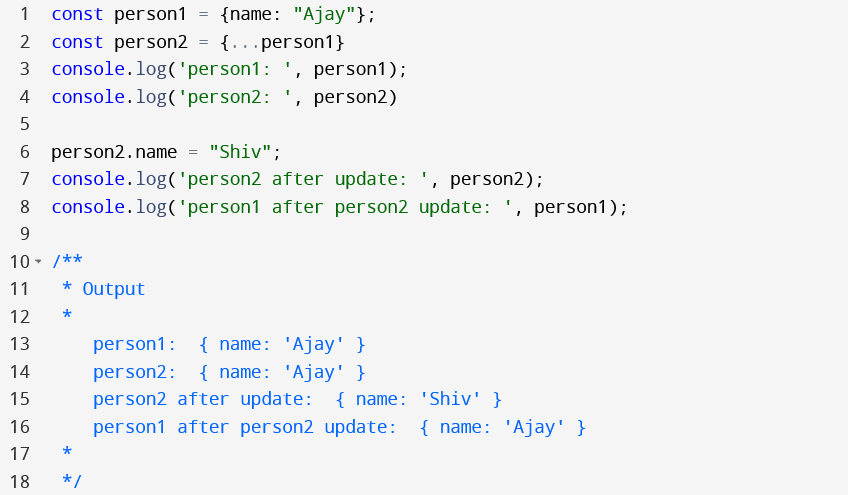
Here on line2 we have used spread syntax and this gives us output as expected.
How to prevent mutation on single object?
In the examples above we were trying to modify the const object. What if you have one requirement where you don’t want your object to reassign some other value? How would you do that?
The solution of the above problem is Object.freeze() method. This method prevents data from being changed easily and original values will be used any time they are accessed across our code

Conclusion
Here we have learned 3 method to prevent object mutation. There can be several other ways which can be used to prevent object mutation. Let me know if you have any concern or if you know any other method in the comment.
Thanks for reading. Happy coding.