
In this blog we will be socket.io in NodeJS application. If you don’t heard of socket.io before
Socket.IO is an event-driven library for real-time web applications. It enables real-time, bi-directional communication between web clients and servers.
You can learn more about it from their official website.
We will be building simple chat application. Here are the points we will be covering
- NodeJS server with socket.io
- Client application listening to socket.io
- Chat Ui and firing socket.io events
Integrate Socket.io with NodeJS application
We will follow below steps to add socket.io in NodeJS application
- First create NodeJS application with the help of express-generator, if you don’t have express generator installed then you can install it by typing following command
npm install -g express-generator
- Now generate NodeJS app with hbs(handlebars) as frontend template.
npx express-generator --view=hbs -d chat
- Above command will create a Node app for you with all required dependencies. Now go to
chatting_app
directory and type following commands
cd chatting_app
npm i
npm start
App will start at port 3000
by default. You can open http://localhost:3000 in your browser.
- Now we will add socket.io in our application. Lets first install socket.io
npm install socket.io --save
- Create a
helper
folder inside project and under helper folder createsocket.helper.js
file and paste below code in this file.
const io = require("socket.io")();
const socketapi = {
io: io
};
// Add your socket.io logic here!
io.on("connection", function (socket) {
console.log("A user connected");
socket.on("message", (data) => {
console.log("message from client", data);
// broadcast received message to all other clients
socket.broadcast.emit("message", {message: "Hello from server: " + data.message})
});
socket.on("end", () => {
console.log("user left");
})
});
// end of socket.io logic
module.exports = socketapi;
- In the above code i am listening to the message event which we will be receiving from the client side. So when we receive message event it will have some data also, we will get that data with the help of callback function.
- Now we will broadcast received message from the client to all other connected clients to the app.
- Now open
bin/www
file and modify it as below
#!/usr/bin/env node
/**
* Module dependencies.
*/
var app = require('../app');
var debug = require('debug')('chatting-app:server');
var http = require('http');
const socketapi = require("../helpers/socket.helper"); // added this line
/**
* Get port from environment and store in Express.
*/
var port = normalizePort(process.env.PORT || '3000');
app.set('port', port);
/**
* Create HTTP server.
*/
var server = http.createServer(app);
socketapi.io.attach(server); // added this line
/**
* Listen on provided port, on all network interfaces.
*/
//...
- In the above code we are attaching socket.io server to NodeJS server.
Now by doing the above changes, you have successfully integrated socket.io in your NodeJS application. Socket.io server is also listening to same port as NodeJS server running.
Add Socket.io on the frontend
First update your layout.hbs file with the following code. Here i have added jQuery, Socket.io client library and custom javascript file in which we are going to write socketIo codes.
<!DOCTYPE html>
<html>
<head>
<title>{{title}}</title>
<link rel='stylesheet' href='/stylesheets/style.css' />
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
</head>
<body>
{{{body}}}
<script src="/socket.io/socket.io.js"></script>
<script src="/javascripts/index.js"></script>
</body>
</html>
Now update index.hbs with the following code. Here i have added one div elements where i will be displaying all messages received from the server and added a input box where we will be typing message.
<div id="main">
<div class="messages"></div>
<input id="message" placeholder="Enter your message">
</div>
Now create index.js file under public/javascripts folder and add below code in it. Here i have added io() constructor which will try to listen to socketIo server by default it will listen to default post and host which is http://localhost:3000.
In the next line i have added two selector 1. for the message input box and 2. messages class selector. On the next line i have added blur event(this event will get fired when an object loses focus) and emitting message to the server.
Now i am listening to the socket.io message event which we are firing on NodeJS backend. In the callback it will have data which we are sending while firing event from the backend.
const socketIo = io();
const message = $("#message"); // input selector on id
const messages = $(".messages"); // messages div selector on class
message.on("blur", function () {
const val = message.val();
// val is not empty
if (val) {
// emit message to server
socketIo.emit('message', { message: val });
}
})
// Receive message from the server
socketIo.on("message", function (data) {
messages.append(`<p>${data.message}</p>`)
})
Here are the two window, when i type anything in one window you will see that message under all other window except current window and vice versa.
Second Window

First Window
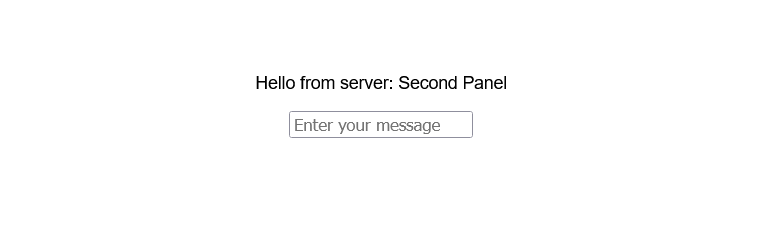
You can open http://localhost:3000 on more than 1 window and try to test it. You will get messages sent from one window to other windows.
Conclusion
In this blog we have seen how we can integrate socket.io to NodeJS application. Here i have taken simple example to build chat application. But you can do lots of good stuff using socket.io like building Notification Feature, Multiplayer Game etc.
Thanks for reading this blog hope you have liked it. If yes then please clap and follow me for more blogs like this. I have my own YouTube channel where i create NodeJS based projects, you can check it out here https://shorturl.at/LMU48. The same blog will also be available on https://ajaykrp.me/