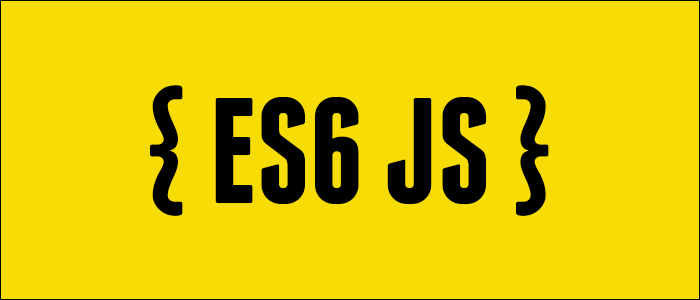
1. Arrow functions
Arrow function is an alternate of javascript normal function. Here you just have to replace function declaration with () => {}. You can pass arguments as well to the arrow functions. You can read more about the difference between arrow function and normal function here.
let arr = [1,2,34,5,6];
arr.forEach((num, idx) => {
console.log(`index(${idx}): ${num}`);
})
2. Block scoping with let
let allows you to declare variables that are limited to the scope of a block statement, or expression on which it is used. So here the output will be 0 1 2 3 4 but if you have used var instead of let then you have got 5 5 5 5 5 as output
for(let i = 0; i <= 4; ++i) {
setTimeout(function () {
console.log(i);
}, 1000);
}
3. Default function value
Now javascript supports default function values the same as other programming languages like Python, Java, etc. So if you haven't provided any value of the default variable then it will pick up the default value else it will pick up the value which you have passed.
function defaultFunction(a, b, c=3) {
return a + b + c;
}
console.log(defaultFunction(3,5));
4. Variadic function arguments
It allows a function to accept an indefinite number of arguments as an array. You can read more here.
// variadic function arguments
function variadicFunctionArgument(a, b, ...nums) {
console.log(nums.reduce((acc, a) => a + acc, 0));
}
variadicFunctionArgument(3, 5, [1,2,4,5,6,7,8]);
5. Spread operator
It allows us to quickly copy all or part of an existing array or objects to another array or objects.
// spread operator
let a = [1,2,4];
let b = [...a, 5, 7];
console.log(b);
6. Object property shorthand
if you want to define an object whose keys have the same name as the variables passed in as properties, you can use the shorthand and simply pass the key name.
// object property shorthand
let c = '555';
let d = '323245'console.log({c,d})
7. Computed object keys
It allows you to dynamically compute the names of the object properties in JavaScript object literal notation.
// computed object keys
let name = 'something';let object = {
[name] : 'somevalue'
}
console.log(object.something);
8. Method notation objects
You can define javascript methods in an object notation format and call methods the same as calling any object variable.
// method notation objectslet methods = {
foo() {
console.log('foo')
},
bar() {
console.log('bar')
}
}
methods.bar()
9. Array Destructuring
Makes it possible to unpack values from arrays, or properties from objects, into distinct.
// array destructinglet arrNew = [1,2,4];
let [one, two, three] = arrNew
console.log(one, two, three)
10. Swap two numbers
// swap two numbers
let x = 5, y = 7;
[x, y] = [y, x];
console.log(x, y)
11. Object Destructuring
It is the same as Array Destructuring.
// object destructing
let objectDesc = {oa: 4, ob: 5};
let {oa, ob} = objectDesc;
console.log(oa, ob);
12. Classes & Getters
The get syntax binds an object property to a function that will be called when that property is looked up.
// classes, gettersclass ClassGetters {
constructor(_id) {
this._id = _id;
}
get id() {
return this._id;
}
}let cg = new ClassGetters(444);
console.log(cg.id);
13. Generators
Function pause execution at a point in the code. Generator functions are written using the function* syntax. When called, generator functions do not initially execute their code. Instead, they return a special type of iterator, called a Generator. When a value is consumed by calling the generator’s next method, the Generator function executes until it encounters the yield keyword.
// generators
function* range(start, end) {
while (start < end) {
yield start; // pause execution
start += 1;
}
}for (let i of range(1, 10)) {
console.log(`generator range: ${i}`);
}
Hope it helps, thanks for reading!