Follow this link to install docker on your machine. I am using Ubuntu so sharing installation guild for Ubuntu https://docs.docker.com/engine/install/ubuntu/ you can find another OS installation guide here as well.
Make sure you have followed the above link correctly to install Docker on your system.
You can type below command to check if docker is running or not
sudo systemctl status docker
You will get following as output

Feel free to ask questions in the comment if you face any difficulties in installation.
Creating NodeJS project
First create one separate directory for this tutorial. I am creating one directory named dockerTest you can also create directory with the same name or you can name anything you like
mkdir dockerTest
Now go to that directory
cd dockerTest
Create one NodeJS project
npm init -y
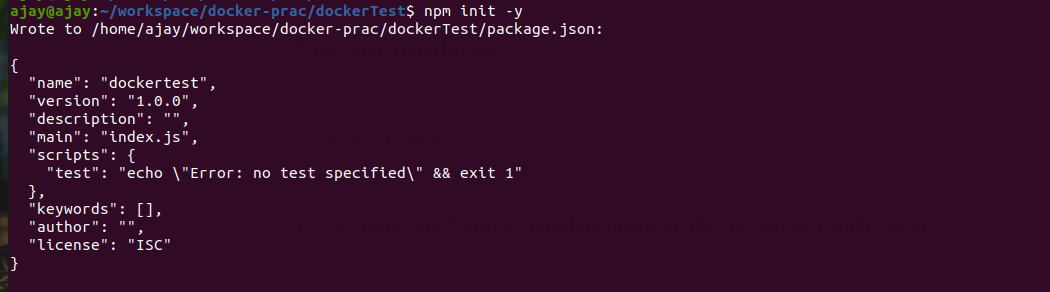
Now lets add start command in package.json, we will be adding index.js as our main file. Your whole package.json file will look like this
{
"name": "dockertest",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "node index.js",
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Now lets install ExpressJS as dependency and create index.js file
npm install express --save
touch index.js
Add below code in index.js file to run Node Server
const express = require("express");
const port = 3000;const app = express();app.get("/", (req, res) => {
res.send("Hello Docker!");
});app.listen(port, () => {
console.log(`App listening to port ${port}`);
})
Now run npm start to check your application is running or not.
Creating Dockerfile for your NodeJS application
A Dockerfile
is a text document that contains all the commands a user could call on the command line to assemble an image. When we tell Docker to build our image by executing the docker build
command, Docker will read these instructions and execute them one by one and create a Docker image as a result.
Let’s walk through creating a Dockerfile
for our application. In the root of your working directory, create a file named Dockerfile
and open this file in your text editor.
FROM node:11-alpine
WORKDIR /appCOPY package.json package.jsonCOPY package-lock.json package-lock.jsonRUN npm installCOPY . .CMD ["npm", "run", "start"]
I will explain you in the detail, what is the meaning of above code
FROM node:11-alpine
Docker images can be inherited from other images. So instead of creating our own base image, we’ll use the official Node.js image that already has all the tools and packages that we need to run a Node.js application. When we use the FROM
command, we tell docker to include in our image all the functionality from the node:11-alpine image
WORKDIR /app
This instructs Docker to use this path as the default location for all subsequent commands. This way we do not have to type out full file paths but can use relative paths based on the working directory.
COPY package.json package.jsonCOPY package-lock.json package-lock.json
Before we can run npm install, we need to get our package.json
and package-lock.json file
s into our images. We’ll use the COPY
command to do this.
RUN npm install
Once we have our package.json files inside the image, we can use the RUN
command to execute the command npm install
. This works exactly the same as if we were running npm install locally on our machine but this time these node modules will be installed into the node_modules directory inside our image.
COPY . .
At this point we have an image that is based on node version 12.18.1 and we have installed our dependencies. The next thing we need to do is to add our source code into the image. We’ll use the COPY command just like we did with our package.json files above.
CMD ["npm", "run", "start"]
This COPY command will take all the files located in the current directory and copies them into the image. Now all we have to do is to tell Docker what command we want to run when our image is run inside of a container. We do this with the CMD command.
Building Images
Now that we’ve created our Dockerfile, let’s build our image. To do this we use the docker build command. The docker build
command builds Docker images from a Dockerfile.
The build command optionally takes a –tag or -t flag. The tag is used to set the name of the image and an optional tag in the format ‘name:tag’. We’ll leave off the optional “tag” for now to help simplify things. If you do not pass a tag, docker will use “latest” as it’s default tag. You’ll see this in the last line of the build output.
Lets build our Docker Image
ajay@ajay ~/w/d/dockerTest [1]> docker build -t node_api .
Sending build context to Docker daemon 2.416MB
Step 1/7 : FROM node:11-alpine
---> f18da2f58c3d
Step 2/7 : WORKDIR /app
---> Running in 0d7a6a65877f
Removing intermediate container 0d7a6a65877f
---> 820672d1fd2a
Step 3/7 : COPY package.json package.json
---> a19fa22366c4
Step 4/7 : COPY package-lock.json package-lock.json
---> 9a1ffd1c7ec6
Step 5/7 : RUN npm install
---> Running in 922d945558b3up to date in 0.862s
found 0 vulnerabilitiesRemoving intermediate container 922d945558b3
---> 18005269ff51
Step 6/7 : COPY . .
---> d72f51689d5d
Step 7/7 : CMD ["npm", "run", "start"]
---> Running in 5b12f51f1331
Removing intermediate container 5b12f51f1331
---> 04da2371794a
Successfully built 04da2371794a
Successfully tagged node_api:latest
Viewing Local Images
To list images, simply run the images
command.
ajay@ajay ~/w/d/dockerTest> docker images
REPOSITORY TAG IMAGE ID CREATED SIZE
node_api latest 04da2371794a 34 minutes ago 77.6MB
node 11-alpine f18da2f58c3d 3 years ago 75.5MB
Running docker container
A container is a normal operating system process except that this process is isolated in that it has its own file system, its own networking, and its own isolated process tree separate from the host.
To run an image inside of a container, we use the docker run command. The docker run
command requires one parameter and that is the image name.
Start the container and expose port 3000 to port 3000 on the host.
ajay@ajay ~/w/d/dockerTest> docker run -p 3000:3000 -t node_api> [email protected] start /app
> node index.jsApp listening to port 3000
Now you can open localhost:3000 and you will see your NodeJS app running.
Thanks for reading. Same post is also available on http://ajaykrp.me/.